by Team Codeasify
1000
A constructor is a special method in classes that is called automatically (we don't have to call this method) when an object of the class is created. In simple terms, it is used to initialize the required attributes of an object at the time of object creation.
If we talk about Python language the constructor is a method with the special name __init__
. Class definitions define this method, which is automatically invoked by python interpreter when an object of the class is created.
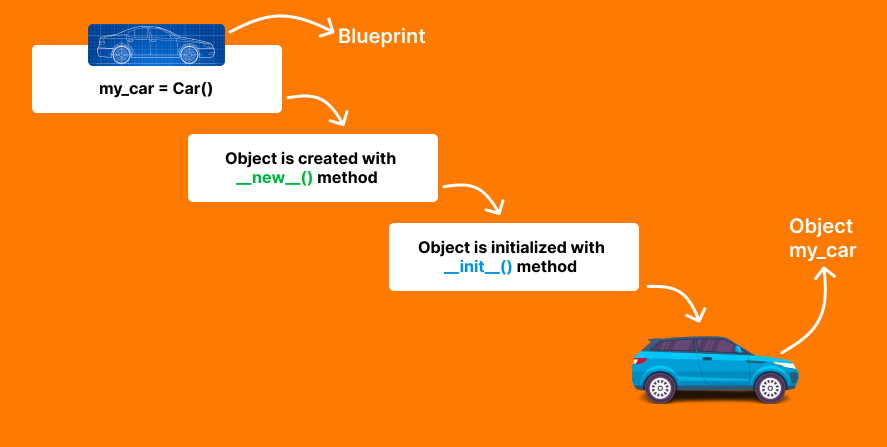
At least one argument must be provided to the __init__
method, self
, it refers to the object that will be created.
To initialize the some other attributes of an object, you can also define additional arguments. We can see that in the below example.
A simple Car class in Python with a constructor looks like this:
class Car:
# constructor method
def __init__(self, company, model, year):
self.company = company
self.model = model
self.year = year
self.speed = 0
This Car class has a constructor __init__
with three arguments: company, model, and year.
The __inti__
method assigns these arguments to the corresponding attributes of the object. It also initializes the speed attribute to 0.
To create an object of the Car class, we can use the Car class as a function and pass in the required attributes like an example given below:
# creating an object of class Car
my_car = Car('Toyota', 'Camry', 2022)
my_car is an object of class Car. Hence a new Car object is created with the company "Toyota", the model "Camry", and the year 2022. Speed is automatically set to 0 when the attribute is created.
Hopefully, this clarifies what a Python constructor is. We would be glad to answer any other questions you may have!