by Team Codeasify
910
Table of Contents
It is important to understand that an object in object-oriented programming (OOP) is a data structure that represents a real-world entity in memory. An entity has both attributes (data/variables) and behaviors (methods/functions) that describe its characteristics and actions.
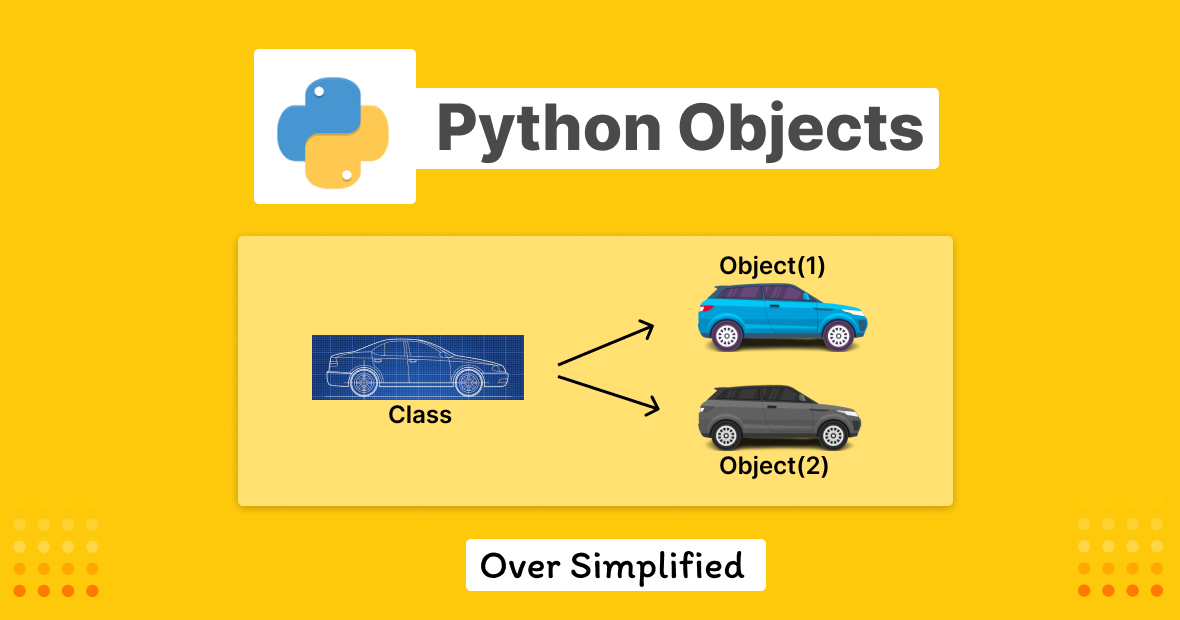
Suppose you have a Car class that defines the attributes and behaviors of a car, such as its model, year, and ability to accelerate and brake. Multiple Car objects could be created to represent specific cars, such as Toyota Camrys and Honda Accords.
How to define a python class
As an example of a simple Python class, here's what it looks like:
class Car:
def __init__(self, company, model, year):
self.company = company
self.model = model
self.year = year
self.speed = 0
def accelerate(self, rate):
self.speed += rate
def brake(self, rate):
self.speed -= rate
This Car class has
Three attributes(variables): company, model, and year.
And two methods(functions): accelerate and brake.
Up until now, we have only defined a class that represents a blueprint/template, not a real-world car entity. In order to create a real car entity, we must create an object for that class.
How to create an object of a python class
To create an object of the Car class, we can use the Car class as a function and pass in the required attributes as given in the below example:
my_car = Car('Toyota', 'Camry', 2020)
This creates a new my_car object with the company "Toyota", model Camry, and year 2020. In the same way, that class can be used to create an unlimited number of objects.
We can access and modify the attributes of the object using dot notation:
print(my_car.make) # Output: Toyota
print(my_car.model) # Output: Camry
print(my_car.year) # Output: 2020
my_car.make = 'Honda'
my_car.model = 'Accord'
my_car.year = 2021
print(my_car.make) # Output: Honda
print(my_car.model) # Output: Accord
print(my_car.year) # Output: 2021
To change the behavior of an object, we can also call its methods:
my_car.accelerate(10)
print(my_car.speed) # Output: 10
my_car.brake(5)
print(my_car.speed) # Output: 5
Using the example of a Car, we hope this clarifies what an object in OOP is.