by Riddhima Singh
8094
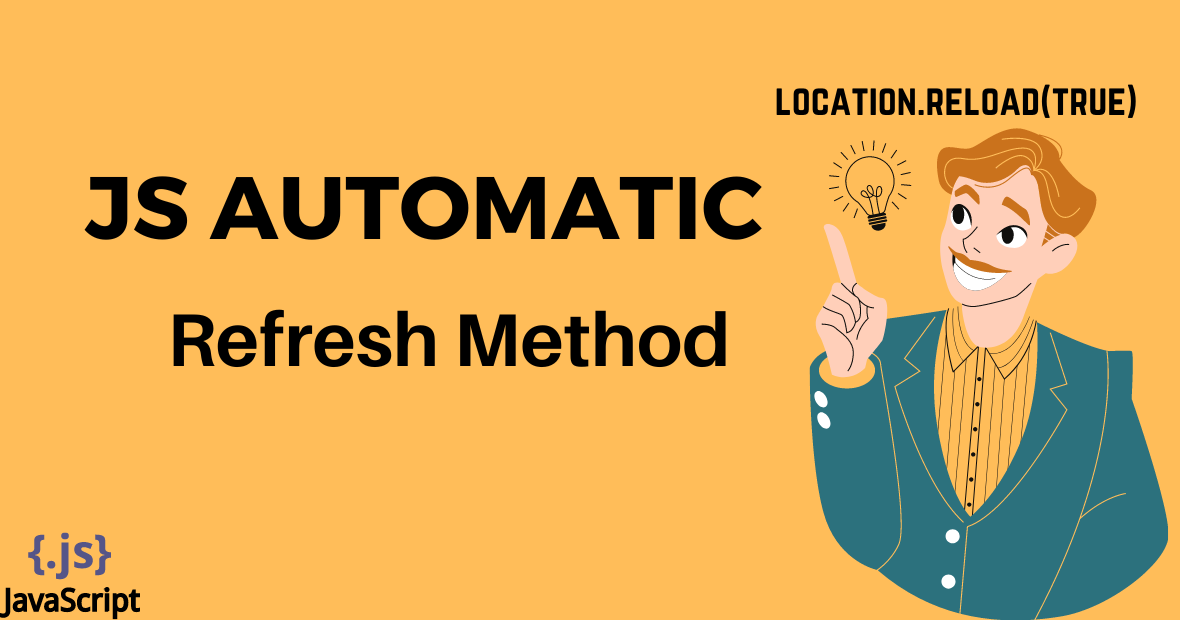
JavaScript location.reload()
, which refreshes a webpage. location.reload(true)
hard-refreshes a page. However, a page can always be reloaded by pressing F5 or clicking the reload button in the browserπ€. location.reload()
makes the browser cache data faster. Images, documents, and data are stored in a browser cache, which is a temporary internal file. But don't forget, the fastest way to refresh a page is to take a deep breath and count to ten... or just yell 'refresh!' really loud π. location.reload()
defaults to 'false'
this parameter requests a server refresh. When false is passed, the webpage is retrieved from the browser's cache.
Syntax
location.reload()
Returns the value
None (undefined)
Working Mechanism of reload() function
When a web page is loaded in a web π browser, the browser stores certain resources (like images, stylesheets, and scripts) in its cache. This caching mechanism improves performance by reusing stored resources when revisiting the page. However, it can sometimes lead to problems, especially when you need to ensure that the page reflects the most up-to-date data from the server.
By using location.reload(true)
, you override the browser's caching mechanism and fetch the page directly from the server. This ensures that any changes made to the server-side content are immediately reflected on the page. Forced reload provides a reliable way to update content dynamically π―.
Code
<!DOCTYPE html>
<html>
<body>
<h1> Hello </h1>
<button onclick = "myFunction( )"> Reload page </button>
<script>
function myFunction( ) {
location.reload ( ) ;
document.write (" Welcome To Codeasify ")
}
</script>
</body>
</html>
Isn't it better to test this function just by trying it and seeing the results π€?
A few common use cases β
π In real-time applications such as stock market prices, news feeds, or chat applications, location.reload(true) can be used to fetch the latest data.
π After submitting a form, it is common to redirect the user to another page or display a success message. Forceful refresh ensures the user can see the changes immediately in scenarios where the form or data has to be displayed on the same page.
π Security and Authentication: It is crucial to ensure that a user's session and permissions are up-to-date when dealing with user authentication and security-sensitive operations. The page will reload right away if you change the authentication status or permissions of the user.
There are some potential downsides to this β
π While `location.reload(true)
` provides a straightforward solution for refreshing a page, it is imperative to consider its potential drawbacks:
π Users may lose unsaved data or be unexpectedly taken from their current position on the page if a forceful page reload occurs frequently.
π Reloading an entire page may increase bandwidth and performance, especially for users with limited internet access or on mobile devices.
Alternatives
There are alternative γ½οΈ techniques for updating specific parts of a web page without reloading the whole page. Ajax (Asynchronous JavaScript and XML)
lets you update specific elements dynamically while making asynchronous requests to the server. You can update data and UI easily with modern JavaScript frameworks like React, Vue.js, and Angular.
Location.reload(true) is deprecated. How can I fix it?
Our solution is to use location.reload() without the force-reload flag instead of location.reload(true)
. It is also possible to check the JavaScript Window Location, which contains information about the current URL.
Conclusion
By default, the JS reload() method reloads the page from the cache. However, you may force it to reload the page from the server side by setting the forceGet parameter
to true : location.reload(true)
.
Hoping you understood the nuances of location.reload(true)
and its applications empower web developers to create dynamic and up-to-date web applications that deliver an exceptional user experience. So, step ahead, and leverage location.reload(true) wisely, and unlock the full potential of your JavaScript projectsπ€©. But remember, with great power comes great responsibility. So, don't use it for evil, or your code may end up reloading...FOREVER π!