by Riddhima Singh
2313
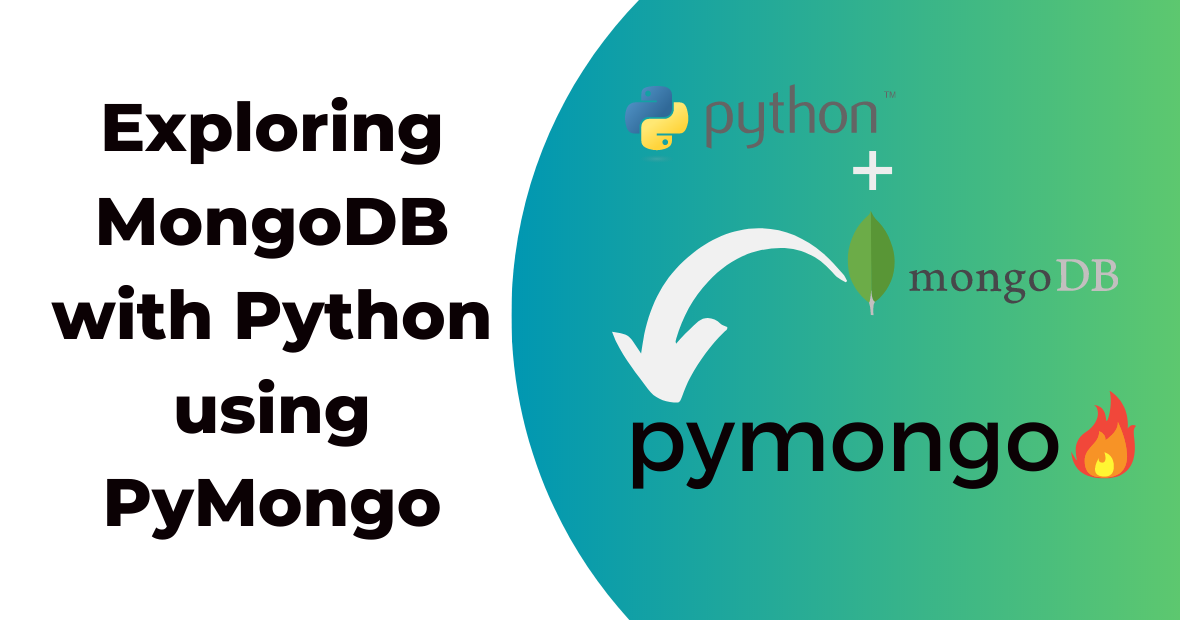
MongoDB is an open-source and document-oriented database. It is classified as a NoSQL database that offers flexibility, scalability, and ease of use for handling and storing data at a very large scale. If you want to use MongoDB with Python then PyMongo
is one of the most popular Python libraries. With PyMongo, you can easily connect to MongoDB, perform database operations, and perform powerful queries. This blog post explores the essential features and functionalities of MongoDB using the PyMongo Python library.
PyMongo makes MongoDB so easy, that even cavemen can do it 😂!
Table of Contents
How to use PyMongo step-by-step
Step 1: Installation of PyMongo
To install PyMongo Generally, we are going to use a Python package manager called pip
. Open the command prompt(For Windows users) or terminal(For Linux users) and use the given 👇 command.
pip install pymongo
Step 2: Establish a connection
To start working with MongoDB, you must connect with the MongoDB server through PyMongo. Start by importing the pymongo
module and using the MongoClient
class to create a connection object.
from pymongo import MongoClient
# Create a MongoDB client
mongodb_server_url = "mongodb://localhost:27017/"
client = MongoClient(mongodb_server_url)
Don't worry if you're not connected, just print client 😴
As MongoDB organizes data into databases containing collections, you can access a database using the connection object and get a reference to a collection within it. There is much to do 🤯:
db = client["mydatabase"]# Access a database
collection = db["mycollection"]# Access a collection
Keep adding these codes in the previous one 😬.
Step 3: Perform CRUD operations
1. Insert a document(Create)
Using the insert_one()
or insert_many()
methods, you can insert a document into a collection.
# Single document insertion
document = {"name": "John", "age": 30}
result = collection.insert_one(document)
print("Inserted document ID:", result.inserted_id)
# Multiple document insertion
documents = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 35}
]
result = collection.insert_many(documents)
print("Inserted document IDs:", result.inserted_ids)
Keep Going We know you can do this 😇...
2. Querying documents(Read)
Here's an example of querying documents using PyMongo based on specific criteria:
# Find documents where age is greater than 30
query = {"age": {"$gt": 30}}
result = collection.find(query)
for document in result:
print(document)
3. Updating document(Update)
You can update MongoDB documents with PyMongo by using the update_one()
or update_many()
methods.
# Update a single document
query = {"name": "John"}
new_values = {"$set": {"age": 32}}
result = collection.update_one(query, new_values)
print("Modified documents:", result.modified_count)
# Update multiple documents
query = {"age": {"$gt": 30}}
new_values = {"$inc": {"age": 1}}
result = collection.update_many(query, new_values)
print("Modified documents:", result.modified_count)
4. Deleting document(Delete)
To delete one or multiple documents, use the delete_one()
or delete_many()
methods:
# Delete a single document
query = {"name": "John"}
result = collection.delete_one(query)
print("Deleted documents:", result.deleted_count)
# Delete multiple documents
query = {"age": {"$gt": 30}}
result = collection.delete_many(query)
print("Deleted documents:", result.deleted_count)
5. Aggregation framework
PyMongo leverages MongoDB's Aggregation Framework by providing a method called aggregate()
:
# Perform aggregation
pipeline = [
{"$group": {"_id": "$name", "count": {"$sum": 1}}}
]
result = collection.aggregate(pipeline)
for document in result:
print(document)
Conclusion
This powerful tool enables seamless integration between Python
and MongoDB
. With an intuitive API, you can insert, query, update, and delete documents using PyMongo
. You can also perform complex data analysis and processing. NoSQL databases can be harnessed to build robust and scalable applications with MongoDB and PyMongo.
You can find more information about PyMongo's capabilities and additional examples in the PyMongo documentation. But if you're in a pinch, you can always just rely on your trusty Google-Fu😂!
Happy Learning 🙂